How TypeScript Enhances Code Safety in React Native
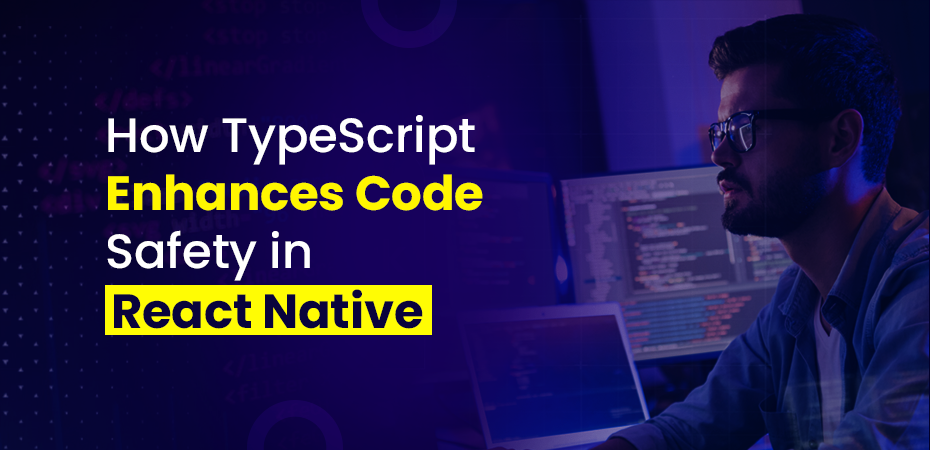
React Native has become a go-to framework for building mobile apps, allowing developers to use JavaScript to create native applications for both Android and iOS. However, working solely with JavaScript can sometimes lead to issues, especially as projects grow in complexity.
This is where TypeScript comes into play. TypeScript, a superset of JavaScript, helps developers catch errors early in the development process, making the code safer and more reliable. In this React Native with TypeScript blog, we’ll explore how TypeScript improves code safety in React Native, focusing on the differences between TSX and JSX and how you can start using TypeScript in your projects.
Understanding TypeScript in React Native
What is TypeScript?
TypeScript is an open-source programming language developed by Microsoft. It builds on JavaScript by adding static type definitions, which means you can define the type of data that a variable can hold. This simple addition helps in preventing many common errors that developers encounter when writing JavaScript code. Whether you’re building a complex feature or something as straightforward as a login and register page in React Native with TypeScript, the addition of TypeScript ensures your code is robust and error-free.
Why Use TypeScript in React Native?
TypeScript offers several benefits that make it a valuable tool in React Native development:
- Catch Errors Early: TypeScript allows you to catch mistakes during the development process instead of at runtime, reducing the number of bugs in your code.
- Improved Code Readability: With TypeScript, your code is more readable and self-documenting because types are explicitly defined.
- Better Tooling Support: Editors and IDEs like Visual Studio Code offer excellent support for TypeScript, providing features like autocomplete, type checking, and intelligent refactoring.
Setting Up TypeScript in a React Native Project
Getting started in a project of React Native with TypeScript is straightforward. Here’s how you can set it up:
- Create a New React Native init Project: Use the command npx react-native init MyApp –template react-native-template-typescript to create a new React Native project with TypeScript enabled.
- Convert an Existing Project: If you have an existing React Native project, you can add TypeScript by installing the required dependencies: npm install typescript @types/react @types/react-native –save-dev.
- Create a tsconfig.json File: This file will configure TypeScript for your project. You can generate it by running npx tsc –init.
React vs. TypeScript: How Do They Work Together?
React and TypeScript are not competing technologies but rather complementary ones. React is a library for building user interfaces, while TypeScript is a language that adds static typing to JavaScript. When used together, they provide a powerful toolset for building robust, scalable, and maintainable applications. React handles the UI logic and rendering, while TypeScript ensures that the code is type-safe and free of common bugs.
TSX vs. JSX – What’s the Difference?
Introduction to JSX
TypeScript JSX, or JavaScript XML, is a syntax extension for JavaScript that looks a lot like HTML. In React, JSX allows you to write what appears to be HTML directly within your JavaScript code, making it easier to build and visualize UI components.
What is TSX?
TSX, or TypeScript XML, is similar to JSX but with TypeScript support. It allows you to write React components with type safety, meaning that you can define and enforce the types of props, state, and other variables within your components.
Comparing TSX and JSX
While JSX and TSX look almost identical, the key difference lies in the type safety that TSX provides. With TSX JS, TypeScript can check your code for type errors, ensuring that your components receive the correct types of props and states. This helps prevent many common bugs that might go unnoticed when using plain JSX.
Key Benefits of TypeScript in React Native Development
1. Type Safety
TypeScript’s most significant advantage is type safety. By defining types for your variables, props, and state, you reduce the likelihood of runtime errors caused by unexpected data types. For example, if a function expects a string but receives a number, TypeScript will throw an error during development, allowing you to fix the issue before it causes problems in production.
Example
interface Props { name: string; age: number; } const Greeting: React.FC<Props> = ({ name, age }) => { return <Text>Hello, my name is {name} and I am {age} years old.</Text>; };
In the above code, if you try to pass a non-string value to name or a non-number value to age, TypeScript will immediately alert you to the mistake.
2. Enhanced Developer Experience
TypeScript enhances the development experience by providing powerful tooling support. When using an editor like Visual Studio Code, TypeScript enables features like:
- Autocomplete: Suggestions based on the defined types and variables in your code.
- Intelligent Code Navigation: Quickly jump to the definition of a variable or function.
- Refactoring Tools: Safely rename variables or functions, with TypeScript ensuring that all instances are correctly updated.
These tools make development faster, more efficient, and less error-prone.
3. Better Code Documentation and Maintenance
TypeScript’s type annotations serve as self-documenting code. When other developers (or even you, after some time) revisit the code, the types help clarify what each function and component is supposed to do. This makes it easier to understand and maintain large codebases, reducing the risk of introducing bugs during updates or refactoring.
4. Interoperability with JavaScript
One of the great things about TypeScript is that it can be gradually adopted in existing JavaScript projects. You don’t have to rewrite your entire codebase in TypeScript. Instead, you can convert one file at a time, allowing you to enjoy the benefits of TypeScript without a massive initial investment. Moreover, TypeScript is fully interoperable with JavaScript, meaning you can continue using your favorite JavaScript libraries and tools.
Practical Examples of TypeScript in React Native
1. Defining Component Props with TypeScript
In React Native, components often receive props, which are inputs that affect how the component behaves or renders. With TypeScript, you can define the type of props a component expects, making your code safer and more predictable.
Example
interface ButtonProps { title: string; onPress: () => void; } const MyButton: React.FC<ButtonProps> = ({ title, onPress }) => { return <Button title={title} onPress={onPress} />; };
Here, the ButtonProps interface defines that title must be a string, and onPress must be a function. If you pass anything else, TypeScript will alert you.
2. State Management with TypeScript
Managing state in React Native is another area where TypeScript shines. By defining the state’s shape, you ensure that all parts of your component work with the correct data types.
Example
const MyComponent: React.FC = () => { const [count, setCount] = useState<number>(0); return ( <View> <Text>{count}</Text> <Button title="Increase" onPress={() => setCount(count + 1)} /> </View> ); };
In this example, TypeScript ensures that count is always a number, preventing potential errors when updating the state.
3. Handling API Responses Safely
When dealing with API responses, it’s crucial to handle the data correctly. TypeScript allows you to define the expected shape of the response, making your code safer.
Example
interface User { id: number; name: string; email: string; } const fetchUser = async (): Promise<User> => { const response = await fetch('https://api.example.com/user'); const data: User = await response.json(); return data; };
By defining a User interface, TypeScript ensures that the API response matches the expected structure, reducing errors when processing the data.
Example: Creating a React Native App Using TypeScript
Let’s walk through the steps to create a React Native app with TypeScript:
- Install React Native CLI: Ensure you have the React Native CLI installed by running npm install -g react-native-cli.
- Create a New Project: Run the following command to create a new project with TypeScript: npx react-native init MyApp –template react-native-template-typescript
- Navigate to Your Project: Use cd MyApp to enter your project directory. Once you’ve set up your project, you can easily get TSX app configurations by ensuring your components are written in TSX files. This ensures your app leverages the full power of TypeScript for type safe React components right from the start.
- Start the Development Server: Run npx react-native run-android or npx react-native run-ios to start your development server.
- Start Coding: Open your project in Visual Studio Code or any code editor of your choice and start building your React Native components using TypeScript.
This is how you can successfully create React App with TypeScript.
Common Challenges and How to Overcome Them
Learning Curve
For developers new to TypeScript, the syntax and concepts might seem challenging at first. However, starting with small projects and gradually introducing TypeScript practice and working with TSX code will help you become more comfortable.
Integration Issues
Integrating TypeScript into existing projects can sometimes be tricky, especially when dealing with third-party libraries that don’t have type definitions. In such cases, you can use TypeScript’s any type as a temporary solution or create custom type definitions.
Type Inference and Declaration Files
When dealing with complex types, you might need to create custom declaration files to help TypeScript understand the types used in your project. While this can be a bit daunting, it ultimately leads to safer and more maintainable code.
Conclusion
TypeScript significantly improves the safety and reliability of React Native applications by catching errors early, enhancing developer experience, and making code easier to maintain. Whether you’re starting a new project or integrating TypeScript into an existing one, the benefits are clear. By using TypeScript in your React Native development, you can build better, more robust applications that stand the test of time.