How to Build a Chat App with React Native and Firebase?
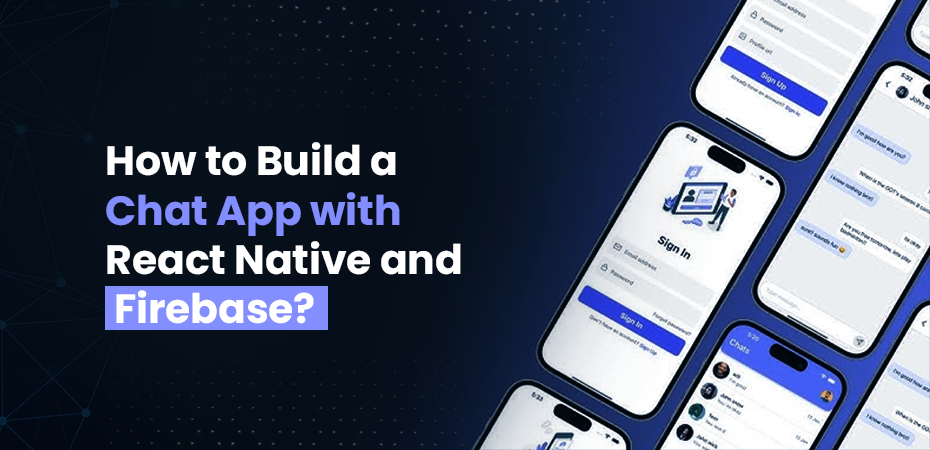
Building a chat app using React Native and Firebase is a rewarding project that combines the power of real-time messaging with an efficient development framework. This tutorial will guide you through the process of creating a fully functional chat application from scratch.
Importance of Real-Time Messaging
Real-time messaging is crucial in modern applications, providing instant communication capabilities that enhance user engagement and satisfaction. Whether for social networking, customer support, or team collaboration, having a reliable chat feature is indispensable.
Key Technologies
- React Native: A popular framework for building cross-platform mobile applications using JavaScript and React.
- Firebase: An all-in-one backend platform from Google that offers services like real-time databases, authentication, and cloud storage.
By combining these technologies, you can create robust chat applications with real-time messaging capabilities, similar to popular apps like WhatsApp.
Prerequisites
Before we start the development process, make sure you have the following things ready:
Necessary Software Installations
Node.js: Ensure you have Node.js installed on your system. This runtime environment allows you to run JavaScript code outside a browser and is crucial for using React Native and various build tools. You can download it from Node.js official website.
Creating a Firebase Account
Firebase Account: Sign up for a Firebase account if you don’t have one already. Navigate to the Firebase Console and create a new project. The Firebase messaging app will serve as the backend for your chats, handling authentication, real-time database updates, and more.
Basic Knowledge Required
JavaScript and React Native
A fundamental understanding of JavaScript and React Native is necessary. Familiarity with these technologies will enable you to navigate through the development process efficiently:
- JavaScript: Understanding ES6+ features such as arrow functions, promises, and async/await.
- React Native: Basic knowledge of components, state management, and lifecycle methods.
Having these prerequisites in place ensures a smoother development experience as we proceed with setting up our project.
Project Setup
Initializing a New React Native Project
To start building your firebase chat app, first, create a new React Native project. Open your terminal and run the following command:
bash npx react-native init ChatApp
This command sets up a new React Native project named ChatApp with the necessary configuration files and folders.
Installing Essential Libraries
Once your project is initialized, navigate to the project directory:
bash cd ChatApp
Next, install the essential libraries for navigation and Firebase integration. Use the following commands to install them:
- React Navigation: bash npm install @react-navigation/native @react-navigation/stack
- Firebase: bash npm install firebase
These libraries are crucial for setting up navigation between screens and connecting your app to Firebase services.
Using Expo for Easier Development
Expo provides tools and services that make developing React Native apps easier. To set up Expo in your existing project, follow these steps:
- First, install the Expo CLI globally if you haven’t already: bash npm install -g expo-cli.
- Initialize a new Expo project: bash expo init ChatAppExpo.
- Choose a template (e.g., blank) and wait for the setup to complete.
Expo simplifies development by offering features like live reloading, an easy setup process, and built-in support for many native APIs.
Summary of Key Points
- Create a New React Native Project: Use npx react-native init ChatApp to start.
- Install Essential Libraries: @react-navigation/native, @react-navigation/stack, and firebase.
- Consider Using Expo: For a streamlined development process with additional tools and services.
By following these steps, you’ll have a solid foundation for making a messaging app using React Native and Firebase.
Setting Up Firebase Configuration
To get started with chat in Firebase, follow these steps:
Setting Up a New Project in the Firebase Console
- Navigate to Firebase Console: Go to the Firebase Console and sign in with your Google account.
- Create a New Project: Click on the “Add project” button. Enter a project name, such as ChatApp, and follow the prompts to configure Google Analytics (optional). Click “Create project” to complete the setup.
- Project Overview: Once the project is created, you will be redirected to the Firebase project dashboard.
Adding a Web App to Link with Your Project
- Register Your App: In the Firebase project dashboard, click on the web icon (</>) to add a new web app. Enter an app nickname and click “Register app”.
- Firebase Configuration Object: After registering your React Native messaging app, you will see a configuration object that looks something like this:
javascript const firebaseConfig = { apiKey: “YOUR_API_KEY”, authDomain: “YOUR_PROJECT_ID.firebaseapp.com”, projectId: “YOUR_PROJECT_ID”, storageBucket: “YOUR_PROJECT_ID.appspot.com”, messagingSenderId: “YOUR_MESSAGING_SENDER_ID”, appId: “YOUR_APP_ID” };
- Copy Configuration Settings: Copy these configuration settings as you will need them to initialize Firebase in your React Native project.
Next, create a firebase.js file in your project’s root directory and paste the configuration object into it:
javascript import firebase from ‘firebase/app’; import ‘firebase/auth’; import ‘firebase/firestore’;
const firebaseConfig = { apiKey: “YOUR_API_KEY”, authDomain: “YOUR_PROJECT_ID.firebaseapp.com”, projectId: “YOUR_PROJECT_ID”, storageBucket: “YOUR_PROJECT_ID.appspot.com”, messagingSenderId: “YOUR_MESSAGING_SENDER_ID”, appId: “YOUR_APP_ID” };
if (!firebase.apps.length) { firebase.initializeApp(firebaseConfig); }
export { firebase };
This setup links your React Native application with Firebase services, making it possible to leverage authentication, Firestore database, and other functionalities.
Creating the Chat App Screens
Designing the Login Screen Interface
To get started, you’ll want to design a user-friendly login screen that allows users to authenticate using Firebase’s authentication methods. Here’s a basic implementation:
1. Create the Login Screen Component:
javascript // src/screens/LoginScreen.js import React, { useState } from ‘react’; import { View, TextInput, Button, StyleSheet } from ‘react-native’; import firebase from ‘../firebase’;
const LoginScreen = ({ navigation }) => { const [email, setEmail] = useState(”); const [password, setPassword] = useState(”);
const handleLogin = () => { firebase.auth().signInWithEmailAndPassword(email, password) .then(() => navigation.navigate(‘Chat’)) .catch(error => alert(error.message)); };
return ( ); };
const styles = StyleSheet.create({ container: { flex: 1, justifyContent: ‘center’, padding: 16, }, input: { height: 40, borderColor: ‘gray’, borderWidth: 1, marginBottom: 12, paddingHorizontal: 8, }, });
export default LoginScreen;
2. Configure Navigation to Chat Screen:
Ensure you have set up navigation properly so that successful login redirects users to the chat interface.
Constructing the Chat Interface
Next, let’s construct an intuitive chat interface for sending and receiving messages. Reusable components will help maintain a clean and modular code structure.
1. Create the Chat Screen Component:
javascript // src/screens/ChatScreen.js import React, { useState, useEffect } from ‘react’; import { GiftedChat } from ‘react-native-gifted-chat’; import firebase from ‘../firebase’;
const ChatScreen = () => { const [messages, setMessages] = useState([]);
useEffect(() => { const unsubscribe = firebase.firestore() .collection(‘chats’) .orderBy(‘createdAt’, ‘desc’) .onSnapshot(querySnapshot => { const messagesFirestore = querySnapshot.docs.map(doc => { const firebaseData = doc.data();
const data = {
_id: doc.id,
text: ”,
createdAt: new Date().getTime(),
…firebaseData,
};
return data;
});
setMessages(messagesFirestore);
});
return () => unsubscribe();
}, []);
const handleSend = async (newMessages) => { const text = newMessages[0].text;
await firebase.firestore().collection(‘chats’).add({
text,
createdAt: new Date().getTime(),
user: {
_id: 1,
name: ‘User Test’,
},
});
setMessages(GiftedChat.append(messages, newMessages));
};
return ( <GiftedChat messages={messages} onSend={(newMessages) => handleSend(newMessages)} user={{ _id: 1 }} /> ); };
export default ChatScreen;
2. Implement Reusable Components:
Utilize reusable components like MessageBubble, InputToolbar, etc., for maintaining a well-structured codebase. Libraries such as react-native-gifted-chat provide built-in customizable components that enhance functionality and UI consistency.
By designing a robust login screen and a functional chat interface, you’re laying down a solid foundation for your chat application. These screens serve as key interaction points for users, enabling seamless communication and authentication within your app.
Implementing Chat Functionality with Firestore Database
To enable real-time messaging in your chat app, leveraging the Firestore Database is essential due to its efficient data storage and real-time update capabilities. Here’s how you can effectively use Firestore for storing chat messages:
Storing Chat Messages
First, set up a Firestore collection to store your chat messages. Each message in the chat will be a document within this collection. A typical structure for a message document might include:
- text: The content of the message.
- createdAt: Timestamp of when the message was created.
- userId: The ID of the user who sent the message.
javascript // Initialize Firestore import firebase from ‘firebase/app’; import ‘firebase/firestore’;
const db = firebase.firestore();
// Function to send a new message const sendMessage = async (message, userId) => { try { await db.collection(‘messages’).add({ text: message, createdAt:
firebase.firestore.FieldValue.serverTimestamp(), userId: userId }); } catch (error) { console.error(“Error adding message: “, error); } };
Setting Up Listeners for Real-Time Updates
Firestore’s real-time listeners allow your app to react immediately to changes in the database. This is crucial for maintaining an up-to-date chat interface.
You can set up a listener on the messages collection to fetch new messages as they are added:
javascript // Listening for real-time updates const listenForMessages = (callback) => { return db.collection(‘messages’) .orderBy(‘createdAt’) .onSnapshot(snapshot => { const messages = snapshot.docs.map(doc => ({ id: doc.id, …doc.data() })); callback(messages); }); };
// Example usage – setting up the listener in a component useEffect(() => { const unsubscribe = listenForMessages((newMessages) => { setMessages(newMessages); });
// Clean up listener on unmount
return () => unsubscribe();
}, []);
Handling Timestamps
When you send a new message, it’s important to include a timestamp. Firestore provides server-side timestamps which ensure consistency across different clients.
javascript // Adding timestamp when sending a message await db.collection(‘messages’).add({ text: newMessage, createdAt:
firebase.firestore.FieldValue.serverTimestamp(), userId: currentUser.id });
Using these methods ensures that your chat app remains responsive and updates in real-time as users send and receive messages.
Managing User Authentication Sessions with Firebase Methods
Implementing user authentication is a crucial part of building any chat application. With Firebase Authentication methods, this process becomes seamless and secure.
User Registration and Login Flow
Start by setting up the user registration and login flow:
1. User Registration
Create a form where users can enter their email and password to sign up. Use Firebase’s createUserWithEmailAndPassword method to handle registration.
javascript firebase.auth().createUserWithEmailAndPassword(email, password) .then((userCredential) => { // Signed in var user = userCredential.user; // … }) .catch((error) => { var errorCode = error.code; var errorMessage = error.message; // Handle Errors here. });
2. User Login
Implement a login form that takes an email and password. Use signInWithEmailAndPassword for authentication.
javascript firebase.auth().signInWithEmailAndPassword(email, password) .then((userCredential) => { // Signed in var user = userCredential.user; // … }) .catch((error) => { var errorCode = error.code; var errorMessage = error.message; // Handle Errors here. });
Managing User Sessions
Managing user sessions is essential for maintaining state across the application. Utilize context or state management libraries like Redux to handle session management effectively.
Using Context API for Session Management
- Create an Auth Context: Set up a context to manage authentication state.
javascript import React, { createContext, useState, useEffect } from ‘react’; import firebase from ‘firebase/app’; import ‘firebase/auth’;
export const AuthContext = createContext();
export const AuthProvider = ({ children }) => { const [user, setUser] = useState(null);
useEffect(() => { const unsubscribe = firebase.auth().onAuthStateChanged((user) => { if (user) { setUser(user); } else { setUser(null); } });
return () => unsubscribe();
}, []);
return ( <AuthContext.Provider value={{ user }}> {children} </AuthContext.Provider> ); };
- Use Auth Context in Components: Access the authentication state within your components using the useContext hook.
javascript import React, { useContext } from ‘react’; import { AuthContext } from ‘./AuthProvider’;
const ChatScreen = () => { const { user } = useContext(AuthContext);
if (!user) { return ; }
return ( {/* Chat UI Here */} Welcome, {user.email} ); };
By integrating Firebase Authentication methods and managing user sessions properly, you ensure a smooth and secure experience for users interacting with your chat app built using React Native and Firebase.
Enhancing Chat UI Components Using react-native-gifted-chat Library
react-native-gifted-chat is an excellent library designed to streamline the development of chat interfaces in React Native applications. Leveraging this chat React library, you can significantly reduce the effort required to build a polished, user-friendly chat UI.
Key Features of react-native-gifted-chat
1. Message Display
The React chat library provides built-in components that handle message rendering efficiently. Messages appear in a bubble format with support for different styles and alignments based on sender and receiver.
2. Input Toolbar
An input toolbar is available out-of-the-box, allowing users to type and send messages seamlessly. It includes features like placeholder text, send button customization, and attachment options.
3. Avatar Support
Easily display user avatars next to messages. You can customize avatar images and sizes to fit your application’s design requirements.
Getting Started with react-native-gifted-chat
1. Installation
Begin by installing the library:
bash npm install react-native-gifted-chat
2. Basic Usage
Import and use the GiftedChat component in your chat screen:
jsx import React, { useState } from ‘react’; import { GiftedChat } from ‘react-native-gifted-chat’;
const ChatScreen = () => { const [messages, setMessages] = useState([]);
const onSend = (newMessages = []) => { setMessages(GiftedChat.append(messages, newMessages)); };
return ( <GiftedChat messages={messages} onSend={newMessages => onSend(newMessages)} user={{ _id: 1, }} /> ); };
export default ChatScreen;
3. Customization
Enhance user experience by customizing various parts of the chat interface:
- Message Bubbles: Customize colors, fonts, and other styles.
jsx <GiftedChat … renderBubble={(props) => ( <Bubble {…props} wrapperStyle={{ right: { backgroundColor: ‘#0084ff’ }, left: { backgroundColor: ‘#e5e5ea’ }, }} /> )} />
Input Toolbar: Modify the appearance and functionality of the input field.
jsx <GiftedChat … renderInputToolbar={(props) => ( <InputToolbar {…props} containerStyle={{ borderTopWidth: 1.5, borderTopColor: ‘#333’ }} /> )} />
Benefits
Using react-native-gifted-chat allows for rapid development while maintaining a high-quality user interface. The customizable components support various visual styles, ensuring that the chat experience aligns with your firebase chat application’s branding.
By integrating this library into your project, you can focus more on backend functionalities and business logic rather than spending excessive time on UI development.
Implementing Navigation Between Screens in React Native App Using react-navigation Library
Smooth navigation is crucial for a seamless user experience in any mobile application. For a chat app built with React Native, using the react-navigation library simplifies the process of navigating between different screens.
Installing react-navigation
To get started, install the necessary packages:
bash npm install @react-navigation/native @react-navigation/stack
Additionally, you need to install the required dependencies for React Native:
bash npm install react-native-screens react-native-safe-area-context
Setting Up Navigation Structure
After installing the required packages, set up your navigation structure. Create a navigation directory and add a MainStackNavigator.js file:
javascript // navigation/MainStackNavigator.js import { createStackNavigator } from ‘@react-navigation/stack’; import { NavigationContainer } from ‘@react-navigation/native’; import LoginScreen from ‘../screens/LoginScreen’; import ChatScreen from ‘../screens/ChatScreen’;
const Stack = createStackNavigator();
const MainStackNavigator = () => { return ( <Stack.Navigator initialRouteName=”Login”> <Stack.Screen name=”Login” component={LoginScreen} /> <Stack.Screen name=”Chat” component={ChatScreen} /> </Stack.Navigator> ); };
export default MainStackNavigator;
Modifying App Entry Point
Ensure your app’s entry point uses the newly created navigator. Update App.js:
javascript // App.js import React from ‘react’; import MainStackNavigator from ‘./navigation/MainStackNavigator’;
const App = () => { return ; };
export default App;
Navigation Between Screens
Implementing navigation within components allows users to transition between the login and chat screens. For example, in LoginScreen.js, navigate to ChatScreen upon successful login:
javascript // screens/LoginScreen.js import React, { useState } from ‘react’; import { View, Button, TextInput } from ‘react-native’; import { useNavigation } from ‘@react-navigation/native’; import firebase from ‘../firebase’;
const LoginScreen = () => { const [email, setEmail] = useState(”); const [password, setPassword] = useState(”); const navigation = useNavigation();
const handleLogin = async () => { try { await firebase.auth().signInWithEmailAndPassword(email, password); navigation.navigate(‘Chat’); } catch (error) { console.error(error); } };
return ( ); };
export default LoginScreen;
The above steps outline how to effectively integrate the react-navigation library into your React Native chat app. This ensures users can smoothly transition between different screens such as login and chat interfaces, enhancing overall usability.
Running Your Chat Application on Android/iOS Devices or Emulators
Running your React JS chat application on physical devices or emulators is a crucial step to ensure everything functions correctly. Whether you’re using an Android or iOS device, following these steps will help you get started:
For Android Devices/Emulators
Set Up Environment:
- Ensure you have Android Studio installed.
- Install the necessary SDKs and create an Android Virtual Device (AVD) if you plan to use an emulator.
Run the Application:
- Connect your physical device via USB or start your emulator through Android Studio.
- Use the command: sh npx react-native run-android
- This command compiles the app and installs it on the connected device/emulator.
For iOS Devices/Simulators
Set Up Environment:
- Ensure Xcode is installed on your Mac.
- Create an iOS simulator through Xcode if you plan to use a virtual device.
Run the Application:
- Connect your physical device via USB or start your simulator through Xcode.
- Use the command: sh npx react-native run-ios
- This command compiles the app and installs it on the connected device/simulator.
Troubleshooting Common Issues
- Error: “Could not connect to development server”: Ensure that both your development machine and device are on the same network.
- Build Failed Errors: Check that all dependencies are correctly installed and configured. Run npm install to re-install node modules if necessary.
- App Not Starting: Ensure that Metro Bundler is running in a separate terminal window using: sh npx react-native start
By carefully following these steps, you’ll be able to see your ChatApp built with React Native and Firebase in action on your chosen devices.
Conclusion
Creating a chat app with React Native and Firebase opens the door to endless possibilities. With the core features established, you can easily expand your app by incorporating additional functionalities.
Building a chat app using React Native and Firebase provides a robust foundation for real-time communication. The skills and knowledge gained through this project are invaluable for any modern application development.